On this post i explain how to upload file/image using c# with max file size and filetype validation
File/image upload refers to the process of transmitting a file or image from a client computer to a server over the internet. In C#, file/image upload can be implemented using various approaches, depending on the specific requirements of the application.
One of the most common ways to handle file/image upload in C# is by using the built-in FileUpload
control of the ASP.NET Web Forms framework. The FileUpload
control is a server control that allows users to select a file from their local computer and upload it to the server.
Here’s an example of how to use the FileUpload
control to handle file upload in an ASP.NET Web Forms application:
Page Name FileUpload.aspx.cs
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="FileUpload.aspx.cs" Inherits="FileUpload" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:FileUpload ID="FileUpload1" runat="server" />
<asp:Button ID="Button1" runat="server" Text="Upload" OnClick="Button1_Click" />
</div>
</form>
</body>
</html>
File Name FileUpload.aspx.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
public partial class FileUpload : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
}
protected void Button1_Click(object sender, EventArgs e)
{
string file_name1 = "";
if (FileUpload1.HasFile)
{
if (System.IO.Path.GetExtension(FileUpload1.FileName) == ".jpg" || System.IO.Path.GetExtension(FileUpload1.FileName) == ".png")
{
int fileSize = FileUpload1.PostedFile.ContentLength;
if (fileSize < 1000000)
{
if (!Directory.Exists(Server.MapPath("Img")))
{
Directory.CreateDirectory(Server.MapPath("Img"));
}
Random r = new Random();
int ran = r.Next(1000);
//this file name to store on database
file_name1 = "Img/" + DateTime.Now.ToString().GetHashCode().ToString("x") + ran.ToString() + Path.GetExtension(FileUpload1.PostedFile.FileName);
FileUpload1.SaveAs(Server.MapPath(file_name1));
ScriptManager.RegisterClientScriptBlock(this, this.GetType(), "alertMessage", "alert('Image upload Successfully')", true);
return;
}
else
{
ScriptManager.RegisterClientScriptBlock(this, this.GetType(), "alertMessage", "alert('Image Max File Size 1MB')", true);
return;
}
}
else
{
ScriptManager.RegisterClientScriptBlock(this, this.GetType(), "alertMessage", "alert('Image should be jpg or png')", true);
return;
}
}
}
}
Out Put
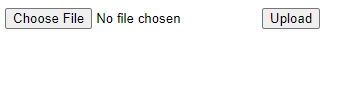