On this blog post we learn about how to change dynamically GridView cell background color based on condition we can also change font color by using this process so lets start
Step 1: first we make a table name of tbl_students and inserts some records
1 2 3 4 5 6 7 8 9 10 11 12 13 | CREATE TABLE [dbo].[tbl_students]( [id] [ int ] NULL , [ name ] [ varchar ](200) NULL , [email_id] [ varchar ](200) NULL , [total_fees] [ int ] NULL , [paid] [ int ] NULL , [dues] [ int ] NULL ) ON [ PRIMARY ] INSERT [dbo].[tbl_students] ([id], [ name ], [email_id], [total_fees], [paid], [dues]) VALUES (1, N 'Rajesh' , N 'rajesh@gmail.com' , 5000, 3000, 2000) INSERT [dbo].[tbl_students] ([id], [ name ], [email_id], [total_fees], [paid], [dues]) VALUES (2, N 'Manoj' , N 'manoj@gmail.com' , 5000, 5000, 0) INSERT [dbo].[tbl_students] ([id], [ name ], [email_id], [total_fees], [paid], [dues]) VALUES (3, N 'Anurg' , N 'anu@gmail.com' , 5000, 5000, 0) INSERT [dbo].[tbl_students] ([id], [ name ], [email_id], [total_fees], [paid], [dues]) VALUES (4, N 'Mohan' , N 'mohan@gmail.com' , 5000, 1000, 4000) |
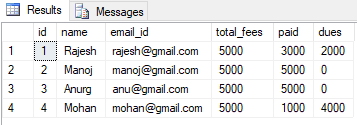
Step 2: create new project on asp.net using C# and create new web form GridView_background.aspx
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 | <%@ Page Language= "C#" AutoEventWireup= "true" CodeBehind= "GridView_background.aspx.cs" Inherits= "CoderRaj.WebForm1" %> <!DOCTYPE html> <head runat= "server" > <title></title> </head> <body> <form id= "form1" runat= "server" > <div> <h1>CoderRaj</h1> <h3>Bind Gridview and change background color based on payment due</h3> <asp:GridView Width= "50%" ID= "GVStudents" runat= "server" ForeColor= "#333333" AutoGenerateColumns= "False" OnRowDataBound= "GVStudents_RowDataBound" > <RowStyle BackColor= "#EFF3FB" /> <Columns> <asp:TemplateField ItemStyle-Width= "10px" HeaderText= "S.N." > <ItemTemplate> <%#Container.DataItemIndex+1 %> </ItemTemplate> </asp:TemplateField> <asp:TemplateField ItemStyle-Width= "150px" HeaderStyle-HorizontalAlign= "Center" HeaderText= "Emp Name" > <ItemTemplate> <asp:Label ID= "lbl_emp_name" runat= "server" Text= '<% #Eval("name") %>' ></asp:Label> </ItemTemplate> <ItemStyle HorizontalAlign= "Center" /> </asp:TemplateField> <asp:TemplateField ItemStyle-Width= "150px" HeaderStyle-HorizontalAlign= "Center" HeaderText= "Email ID" > <ItemTemplate> <asp:Label ID= "lbl_email_id" runat= "server" Text= '<% #Eval("email_id") %>' ></asp:Label> </ItemTemplate> <ItemStyle HorizontalAlign= "Center" /> </asp:TemplateField> <asp:TemplateField ItemStyle-Width= "150px" HeaderStyle-HorizontalAlign= "Center" HeaderText= "Fees" > <ItemTemplate> <asp:Label ID= "lbl_total_fees" runat= "server" Text= '<% #Eval("total_fees") %>' ></asp:Label> </ItemTemplate> <ItemStyle HorizontalAlign= "Center" /> </asp:TemplateField> <asp:TemplateField ItemStyle-Width= "150px" HeaderStyle-HorizontalAlign= "Center" HeaderText= "Paid" > <ItemTemplate> <asp:Label ID= "lbl_paid" runat= "server" Text= '<% #Eval("paid") %>' ></asp:Label> </ItemTemplate> <ItemStyle HorizontalAlign= "Center" /> </asp:TemplateField> <asp:TemplateField ItemStyle-Width= "150px" HeaderStyle-HorizontalAlign= "Center" HeaderText= "Paid" > <ItemTemplate> <asp:Label ID= "lbl_due" runat= "server" Text= '<% #Eval("dues") %>' ></asp:Label> </ItemTemplate> <ItemStyle HorizontalAlign= "Center" /> </asp:TemplateField> </Columns> <FooterStyle BackColor= "#507CD1" Font-Bold= "True" HorizontalAlign= "Center" ForeColor= "black" /> <PagerStyle BackColor= "#2461BF" ForeColor= "White" HorizontalAlign= "Center" /> <SelectedRowStyle BackColor= "#D1DDF1" Font-Bold= "True" ForeColor= "#333333" /> <HeaderStyle BackColor= "#507CD1" Font-Bold= "True" ForeColor= "Black" /> <EditRowStyle BackColor= "#2461BF" /> <AlternatingRowStyle BackColor= "White" /> </asp:GridView><br /> <div style= "height:25px; width:25px; background-color:#AFE1AF" ></div>Fees Paid<br /> <div style= "height:25px; width:25px; background-color:#f2dede" ></div> Fees Due </div> </form> </body> </html> |
Code behind page GridView_background.aspx.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 | using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; using System.Data.SqlClient; using System.Configuration; using System.Data; namespace CoderRaj { public partial class WebForm1 : System.Web.UI.Page { string ConStr; protected void Page_Load( object sender, EventArgs e) { BindStudents(); } public void BindStudents() { try { ConStr = ConfigurationManager.ConnectionStrings[ "CoderRaj_ConStr" ].ConnectionString; SqlDataAdapter Adp = new SqlDataAdapter( "select * from tbl_students" , ConStr); DataTable Dt = new DataTable(); Adp.Fill(Dt); if (Dt.Rows.Count > 0) { GVStudents.DataSource = Dt; GVStudents.DataBind(); } } catch (Exception ex) { } } protected void GVStudents_RowDataBound( object sender, GridViewRowEventArgs e) { if (e.Row.RowType == DataControlRowType.DataRow) { string due = Convert.ToString(DataBinder.Eval(e.Row.DataItem, "paid" )); string total_fees = Convert.ToString(DataBinder.Eval(e.Row.DataItem, "total_fees" )); if (total_fees != due) { e.Row.BackColor = System.Drawing.ColorTranslator.FromHtml( "#f2dede" ); } else { e.Row.BackColor = System.Drawing.ColorTranslator.FromHtml( "#AFE1AF" ); } } } } } |
Step 3: Web.config
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | <?xml version= "1.0" encoding= "utf-8" ?> <!-- For more information on how to configure your ASP.NET application, please visit https: //go.microsoft.com/fwlink/?LinkId=169433 --> <configuration> <connectionStrings> <!--connection string --> <add connectionString= "Data Source =127.0.0.1; Initial Catalog=CODERRAJ;User ID=sa;Password=123;" name= "CoderRaj_ConStr" providerName= "System.Data.SqlClient" /> </connectionStrings> <system.web> <compilation debug= "true" targetFramework= "4.7.2" /> <httpRuntime targetFramework= "4.7.2" /> </system.web> <system.codedom> <compilers> <compiler language= "c#;cs;csharp" extension= ".cs" type= "Microsoft.CodeDom.Providers.DotNetCompilerPlatform.CSharpCodeProvider, Microsoft.CodeDom.Providers.DotNetCompilerPlatform, Version=2.0.1.0, Culture=neutral, PublicKeyToken=31bf3856ad364e35" warningLevel= "4" compilerOptions= "/langversion:default /nowarn:1659;1699;1701" /> <compiler language= "vb;vbs;visualbasic;vbscript" extension= ".vb" type= "Microsoft.CodeDom.Providers.DotNetCompilerPlatform.VBCodeProvider, Microsoft.CodeDom.Providers.DotNetCompilerPlatform, Version=2.0.1.0, Culture=neutral, PublicKeyToken=31bf3856ad364e35" warningLevel= "4" compilerOptions= "/langversion:default /nowarn:41008 /define:_MYTYPE=\"Web\" /optionInfer+" /> </compilers> </system.codedom> </configuration> |
Output
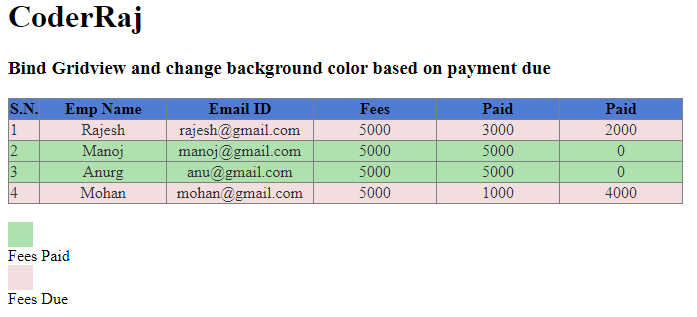