To add Toastr alert on asp.net core first we need to make a partial page so first we need to add a view with the name of _Notificcation (partial page always start with underscore(_))
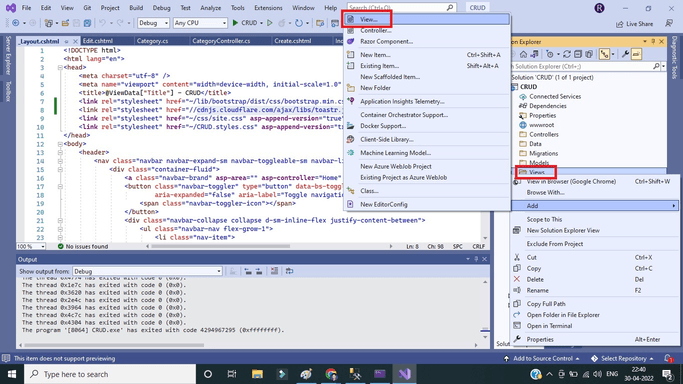
After that past below code on _Notification.cshtml
@if (TempData["success"] != null)
{
<script src="~/lib/jquery/dist/jquery.min.js"></script>
<script src="//cdnjs.cloudflare.com/ajax/libs/toastr.js/latest/toastr.min.js"></script>
<script type="text/javascript">
toastr.success('@TempData["success"]')
</script>
}
@if (TempData["error"] != null)
{
<script src="~/lib/jquery/dist/jquery.min.js"></script>
<script src="//cdnjs.cloudflare.com/ajax/libs/toastr.js/latest/toastr.min.js"></script>
<script type="text/javascript">
toastr.error('@TempData["error"]')
</script>
}
Then add this below line code on your view page ( index. cshtml )where you want to use this alert
<partial name="_Notification" />
Then open _layout.cshtml then add below css
<link rel="stylesheet" href="//cdnjs.cloudflare.com/ajax/libs/toastr.js/latest/toastr.min.css" />
Then add (TempData["success"] = "Category Added Successfully"
) Message on your controller just like below example
public IActionResult Create(Category obj)
{
if(obj.Name== obj.DisplayOrder.ToString())
{
ModelState.AddModelError("CustomError","Display Order and Name are Same");
}
if (ModelState.IsValid)
{
_db.Categories.Add(obj);
_db.SaveChanges();
TempData["success"] = "Category Added Successfully";
return RedirectToAction("Index");
}
return View(obj);
}
Output:-
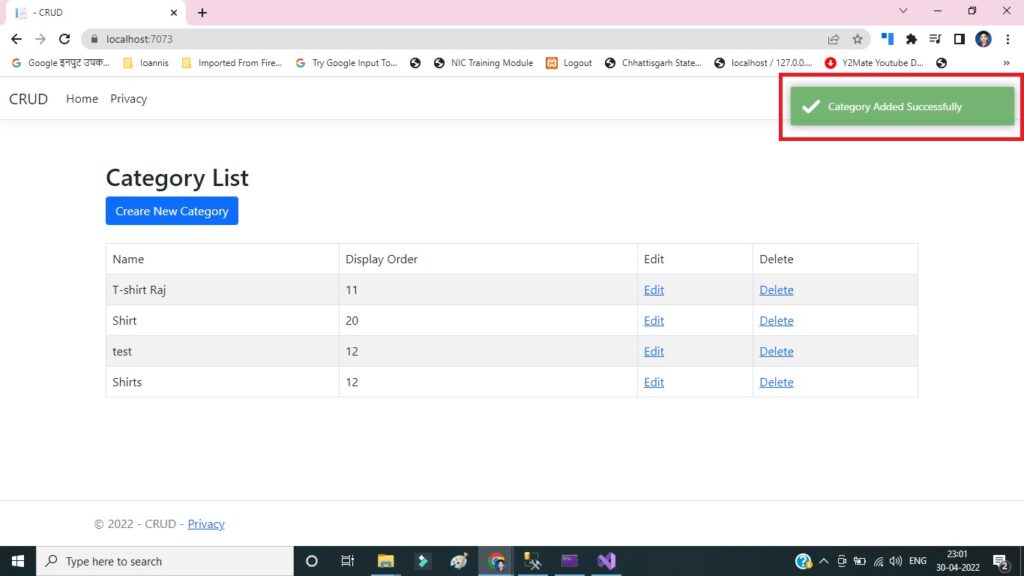
Important link https://github.com/CodeSeven/toastr