On this blog we learn about how to use tooltip inside GridView using C# asp.net below some steps follow
Before we start this blog you have basic knowledge of HTML ,CSS ,SQL Server and C# asp.net.
GridView :- In ASP.NET, the GridView
control is commonly used to display tabular data in a web application. It provides a flexible and customizable way to present data in rows and columns.
Tooltips :- Tooltips provide additional information when users hover over a control.
Step 1:- Step 1: first we make a table name of tbl_students and inserts some records sql query also mention below.
CREATE TABLE [dbo].[tbl_students](
[id] [int] NULL,
[name] [varchar](200) NULL,
[email_id] [varchar](200) NULL,
[total_fees] [int] NULL,
[paid] [int] NULL,
[dues] [int] NULL,
[remark] [varchar](500) NULL
) ON [PRIMARY]
INSERT [dbo].[tbl_students] ([id], [name], [email_id], [total_fees], [paid], [dues], [remark]) VALUES (1, N'Rajesh', N'rajesh@gmail.com', 5000, 3000, 2000, N' join for php class')
INSERT [dbo].[tbl_students] ([id], [name], [email_id], [total_fees], [paid], [dues], [remark]) VALUES (2, N'Manoj', N'manoj@gmail.com', 5000, 5000, 0, N'computer test')
INSERT [dbo].[tbl_students] ([id], [name], [email_id], [total_fees], [paid], [dues], [remark]) VALUES (3, N'Anurg', N'anu@gmail.com', 5000, 5000, 0, N'summer cours')
INSERT [dbo].[tbl_students] ([id], [name], [email_id], [total_fees], [paid], [dues], [remark]) VALUES (4, N'Mohan', N'mohan@gmail.com', 5000, 1000, 4000, N'java class')
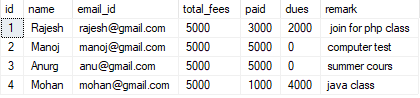
Step 2: create new project on C# asp.net and create new web form GridView_Tooltip.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="GridView_Tooltip.aspx.cs" Inherits="GridView_Tooltip.GridView_Tooltip" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
</head>
<body>
<form id="form1" runat="server">
<div>
<h1>CoderRaj</h1>
<h3>Use Toolip inside Gridview dynamic</h3>
<asp:GridView Width="50%" ID="GVStudents" runat="server"
ForeColor="#333333" AutoGenerateColumns="False" OnRowDataBound="GVStudents_RowDataBound" >
<RowStyle BackColor="#EFF3FB" />
<Columns>
<asp:TemplateField ItemStyle-Width="10px" HeaderText="S.N.">
<ItemTemplate>
<%#Container.DataItemIndex+1 %>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField ItemStyle-Width="150px" HeaderStyle-HorizontalAlign="Center" HeaderText="Emp Name">
<ItemTemplate>
<asp:Label ID="lbl_emp_name" runat="server" Text='<% #Eval("name") %>'></asp:Label>
</ItemTemplate>
<ItemStyle HorizontalAlign="Center" />
</asp:TemplateField>
<asp:TemplateField ItemStyle-Width="150px" HeaderStyle-HorizontalAlign="Center" HeaderText="Email ID">
<ItemTemplate>
<asp:Label ID="lbl_email_id" runat="server" Text='<% #Eval("email_id") %>'></asp:Label>
</ItemTemplate>
<ItemStyle HorizontalAlign="Center" />
</asp:TemplateField>
<asp:TemplateField ItemStyle-Width="150px" HeaderStyle-HorizontalAlign="Center" HeaderText="Fees">
<ItemTemplate>
<asp:Label ID="lbl_total_fees" runat="server" Text='<% #Eval("total_fees") %>'></asp:Label>
</ItemTemplate>
<ItemStyle HorizontalAlign="Center" />
</asp:TemplateField>
<asp:TemplateField ItemStyle-Width="150px" HeaderStyle-HorizontalAlign="Center" HeaderText="Paid">
<ItemTemplate>
<asp:Label ID="lbl_paid" runat="server" Text='<% #Eval("paid") %>'></asp:Label>
</ItemTemplate>
<ItemStyle HorizontalAlign="Center" />
</asp:TemplateField>
<asp:TemplateField ItemStyle-Width="150px" HeaderStyle-HorizontalAlign="Center" HeaderText="Paid">
<ItemTemplate>
<asp:Label ID="lbl_due" runat="server" Text='<% #Eval("dues") %>'></asp:Label>
</ItemTemplate>
<ItemStyle HorizontalAlign="Center" />
</asp:TemplateField>
<asp:TemplateField Visible="false" ItemStyle-Width="150px" HeaderStyle-HorizontalAlign="Center" HeaderText="Remark">
<ItemTemplate>
<asp:Label ID="lbl_due" runat="server" Text='<% #Eval("remark") %>'></asp:Label>
</ItemTemplate>
<ItemStyle HorizontalAlign="Center" />
</asp:TemplateField>
</Columns>
<FooterStyle BackColor="#507CD1" Font-Bold="True" HorizontalAlign="Center" ForeColor="black" />
<PagerStyle BackColor="#2461BF" ForeColor="White" HorizontalAlign="Center" />
<SelectedRowStyle BackColor="#D1DDF1" Font-Bold="True" ForeColor="#333333" />
<HeaderStyle BackColor="#507CD1" Font-Bold="True" ForeColor="Black" />
<EditRowStyle BackColor="#2461BF" />
<AlternatingRowStyle BackColor="White" />
</asp:GridView><br />
</form>
</body>
</html>
Code behind page GridView_Tooltip.aspx.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Data.SqlClient;
using System.Configuration;
using System.Data;
namespace GridView_Tooltip
{
public partial class GridView_Tooltip : System.Web.UI.Page
{
string ConStr;
protected void Page_Load(object sender, EventArgs e)
{
BindStudents();
}
public void BindStudents()
{
try
{
ConStr = ConfigurationManager.ConnectionStrings["CoderRaj_ConStr"].ConnectionString;
SqlDataAdapter Adp = new SqlDataAdapter("select * from tbl_students", ConStr);
DataTable Dt = new DataTable();
Adp.Fill(Dt);
if (Dt.Rows.Count > 0)
{
GVStudents.DataSource = Dt;
GVStudents.DataBind();
}
}
catch (Exception ex)
{
}
}
protected void GVStudents_RowDataBound(object sender, GridViewRowEventArgs e)
{
if (e.Row.RowType == DataControlRowType.DataRow)
{
string remark = Convert.ToString(DataBinder.Eval(e.Row.DataItem, "remark"));
//show tooltip when mouse over on name
e.Row.Cells[1].ToolTip = remark;
}
}
}
}
Step 3: Web.config connection string details
<?xml version="1.0" encoding="utf-8"?>
<!--
For more information on how to configure your ASP.NET application, please visit
https://go.microsoft.com/fwlink/?LinkId=169433
-->
<configuration>
<connectionStrings>
<!--connection string-->
<add connectionString="Data Source =127.0.0.1; Initial Catalog=CODERRAJ;User ID=sa;Password=123;" name="CoderRaj_ConStr" providerName="System.Data.SqlClient" />
</connectionStrings>
<system.web>
<compilation debug="true" targetFramework="4.7.2" />
<httpRuntime targetFramework="4.7.2" />
</system.web>
<system.codedom>
<compilers>
<compiler language="c#;cs;csharp" extension=".cs" type="Microsoft.CodeDom.Providers.DotNetCompilerPlatform.CSharpCodeProvider, Microsoft.CodeDom.Providers.DotNetCompilerPlatform, Version=2.0.1.0, Culture=neutral, PublicKeyToken=31bf3856ad364e35" warningLevel="4" compilerOptions="/langversion:default /nowarn:1659;1699;1701" />
<compiler language="vb;vbs;visualbasic;vbscript" extension=".vb" type="Microsoft.CodeDom.Providers.DotNetCompilerPlatform.VBCodeProvider, Microsoft.CodeDom.Providers.DotNetCompilerPlatform, Version=2.0.1.0, Culture=neutral, PublicKeyToken=31bf3856ad364e35" warningLevel="4" compilerOptions="/langversion:default /nowarn:41008 /define:_MYTYPE=\"Web\" /optionInfer+" />
</compilers>
</system.codedom>
</configuration>
Output
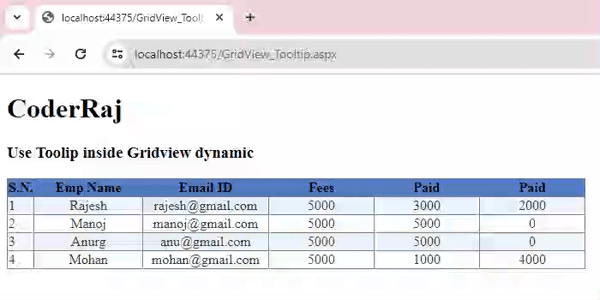